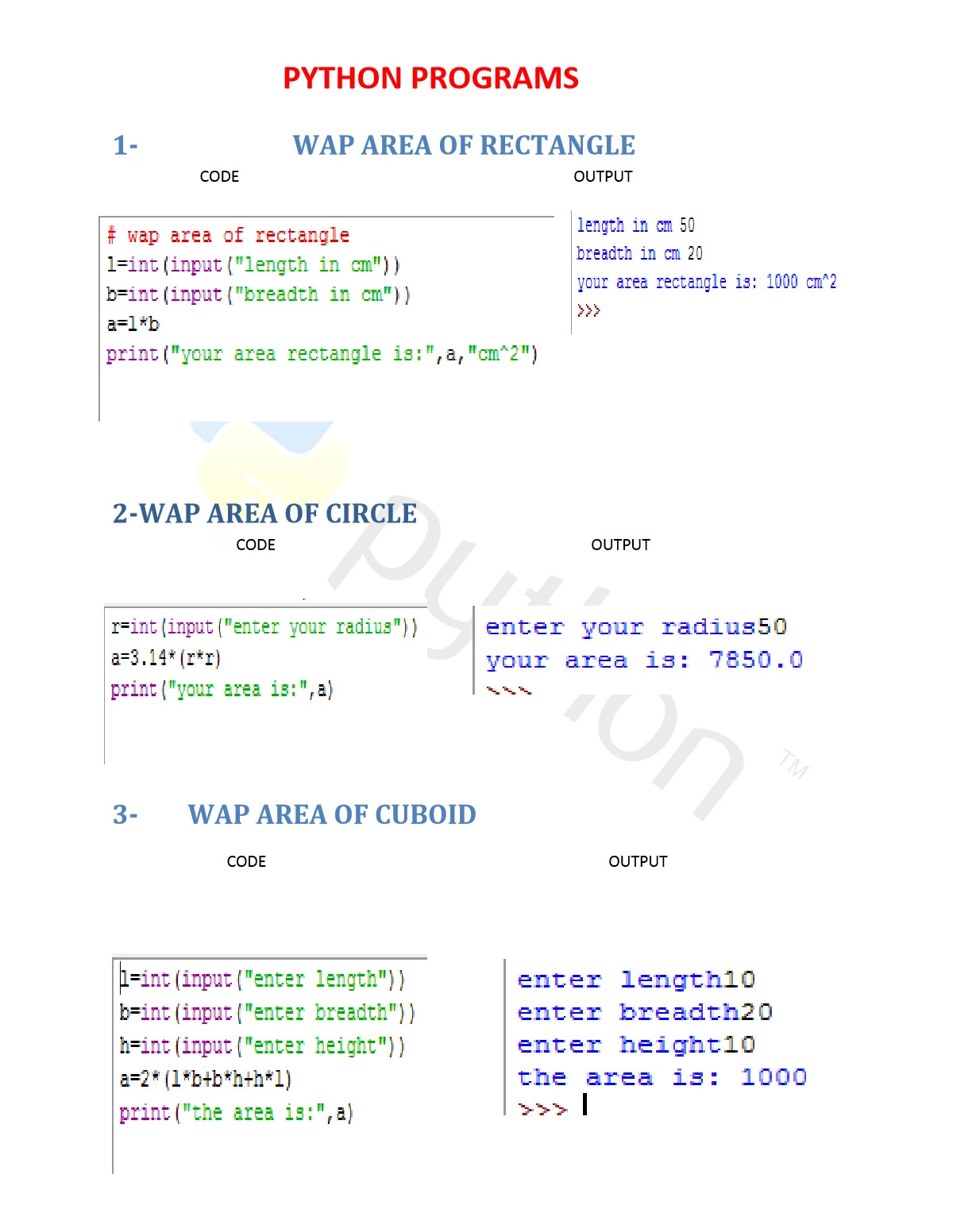
List of Python Programs with Solutions
A Python program is a script or a set of instructions written in the Python programming language. Python is a high-level, interpreted language known for its simplicity and readability. It is widely used in various fields such as web development, data analysis, artificial intelligence, scientific computing, and more.
List of Python Programs
Program Description | Sample Code |
---|---|
Hello, World! | print("Hello, World!") |
Addition of Two Numbers | python<br>def add(a, b):<br> return a + b<br><br>result = add(5, 3)<br>print("Sum:", result) |
Factorial of a Number | python<br>def factorial(n):<br> if n == 0:<br> return 1<br> else:<br> return n * factorial(n-1)<br><br>print("Factorial of 5:", factorial(5)) |
Fibonacci Sequence | python<br>def fibonacci(n):<br> sequence = [0, 1]<br> while len(sequence) < n:<br> sequence.append(sequence[-1] + sequence[-2])<br> return sequence<br><br>print("First 10 Fibonacci numbers:", fibonacci(10)) |
Check Prime Number | python<br>def is_prime(num):<br> if num <= 1:<br> return False<br> for i in range(2, int(num ** 0.5) + 1):<br> if num % i == 0:<br> return False<br> return True<br><br>print("Is 7 prime?", is_prime(7)) |
Reverse a String | python<br>def reverse_string(s):<br> return s[::-1]<br><br>print("Reverse of 'hello':", reverse_string("hello")) |
Find Largest Element in List | python<br>def find_largest(lst):<br> return max(lst)<br><br>print("Largest element:", find_largest([1, 2, 3, 4, 5])) |
Count Vowels in a String | python<br>def count_vowels(s):<br> vowels = "aeiou"<br> return sum(1 for char in s if char.lower() in vowels)<br><br>print("Vowels in 'hello':", count_vowels("hello")) |
Sum of Digits in a Number | python<br>def sum_of_digits(n):<br> return sum(int(digit) for digit in str(n))<br><br>print("Sum of digits in 123:", sum_of_digits(123)) |
Bubble Sort Algorithm | python<br>def bubble_sort(arr):<br> n = len(arr)<br> for i in range(n):<br> for j in range(0, n-i-1):<br> if arr[j] > arr[j+1]:<br> arr[j], arr[j+1] = arr[j+1], arr[j]<br> return arr<br><br>print("Sorted list:", bubble_sort([64, 34, 25, 12, 22, 11, 90])) |